要使用EF Core 連線MongoDB,我們首先要安裝以下套件
- MongoDB.EntityFrameworkCore
到方案總管的相依性>管理NuGet套件
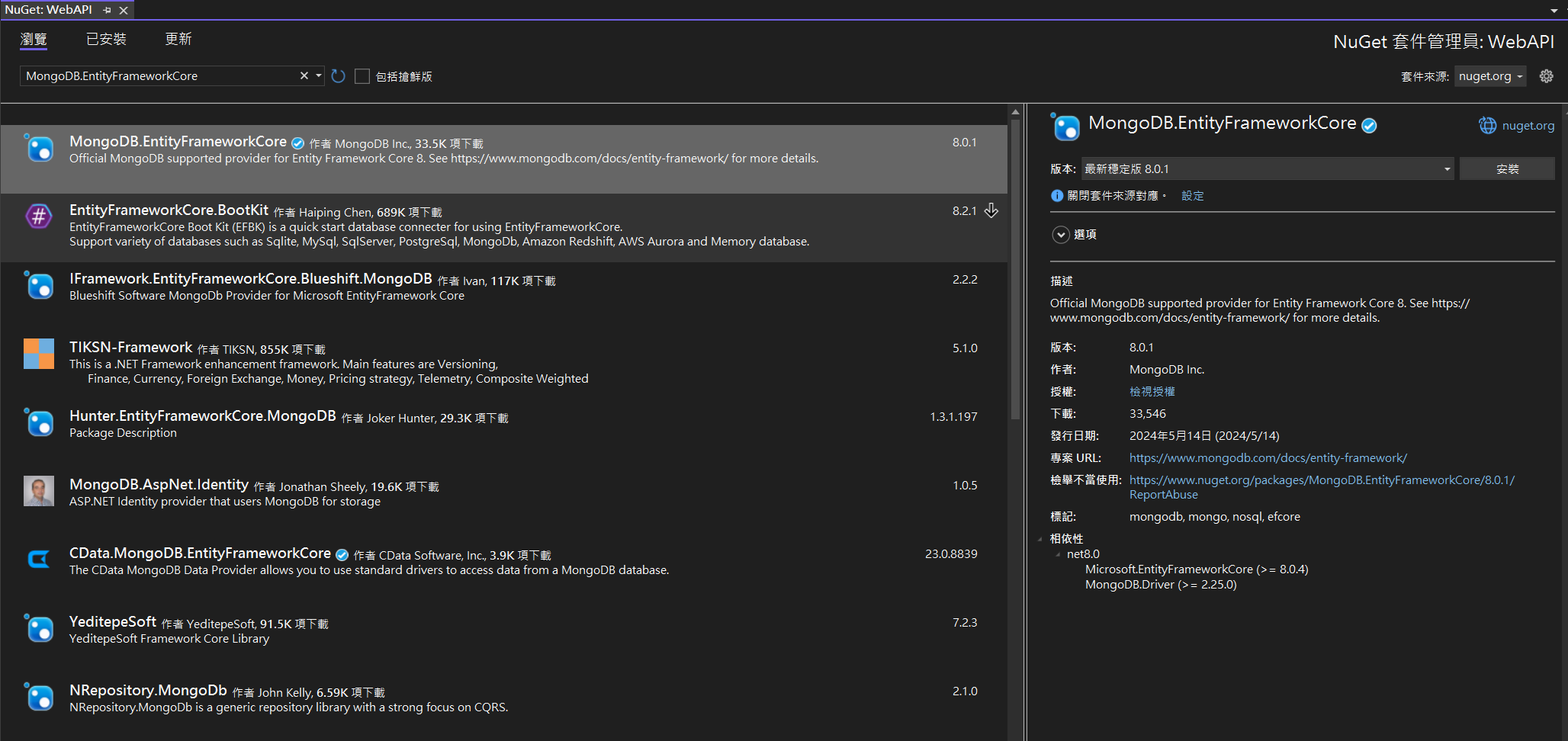
如果有看過前面的人,這邊不用裝Microsoft.EntityFrameworkCore.Tools,因為MongoDB不是關聯式資料庫
而這個工具是要用在關聯式資料庫產生相關的物件或建立資料庫資料表用的,所以這邊用不到
而對應的資料表類別,則要自己建立出來,沒有工具可以幫忙
接著我們建立一個Models資料夾,並在下面新增一個類別,這是對應你資料庫的資料表檔案
假使我們有一個資料表要取名為News,那我們就建立一個News的class

接著在裡面打上對應的欄位名稱
using MongoDB.Bson;
using MongoDB.Bson.Serialization.Attributes;
namespace WebAPI.Models
{
public class News
{
[BsonId]
[BsonRepresentation(BsonType.ObjectId)]
public string _id { get; set; }
public string title { get; set; }
public string content { get; set; }
}
}
這邊_id比較特別需要放上特別的標籤[BsonRepresentation(BsonType.ObjectId)],後續才能夠正常對應MongoDB的資料表_id
接著我們再建立一個類別WebDatabaseSettings.cs,這是等等要用的設定檔為了有強型別用的
namespace WebAPI.Models
{
public class WebDatabaseSettings
{
public string ConnectionString { get; set; }
public string DatabaseName { get; set; }
public string NewsCollectionName { get; set; }
}
}
再來一樣在我們的appsettings.json,放上MongoDB的連線字串參數
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*",
"WebDatabase": {
"ConnectionString": "mongodb://Web:123456@localhost:27017/?authSource=Web",
"DatabaseName": "Web",
"NewsCollectionName": "News"
}
}
都設定好了之後,這邊一樣到Program.cs設定依賴注入,但是這邊只是注入參數而已,不是資料庫物件
builder.Services.Configure<WebDatabaseSettings>(builder.Configuration.GetSection("WebDatabase"));
以上都設定完了之後,我們就可以建立NewsController.cs,來試著能不能從MongoDB取出資料了
namespace WebAPI.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class NewsController : ControllerBase
{
private readonly IMongoCollection<News> _newsCollection; //宣告要使用的資料表
public NewsController(IOptions<WebDatabaseSettings> webDatabaseSettings) //這邊是依賴注入取得相關連線參數
{
var mongoClient = new MongoClient(
webDatabaseSettings.Value.ConnectionString); //設定MongoDB連線字串
var mongoDatabase = mongoClient.GetDatabase(
webDatabaseSettings.Value.DatabaseName); //設定要從哪個資料庫取資料
_newsCollection = mongoDatabase.GetCollection<News>(
webDatabaseSettings.Value.NewsCollectionName); //設定要從哪個資料表取資料,並給上方宣告的全域變數
}
}
}
之後就可以來取出News裡的資料了
[HttpGet]
public IEnumerable<News> Get()
{
return _newsCollection.Find(a => true).ToList();
}
最後按下啟動不偵錯,看看我們建立的三筆資料有沒有建立上去且成功被撈出來。
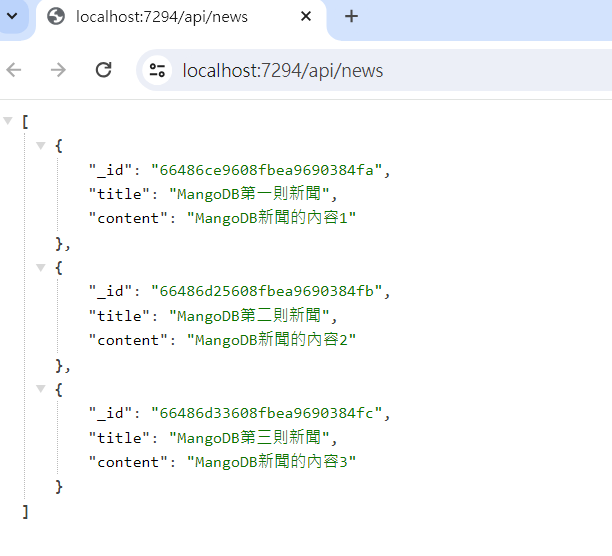
上圖資料都撈出來了,就代表我們這次的設定是沒有問題的
這邊只是起個頭簡單示範如何從MongoDB取出資料,以後還想知道其他用法,就要靠大家自己去學習了
畢竟我沒在使用MongoDB,一些相關的應用並不熟練XD